Monochrome Effects
TeshFx provides several “filters”. A filter here is an effect applied to an image, and the original image can be changed in various ways by the filter.
In this article, we will discuss the “TeshFxMonoColorFilter,” a filter that turns a color image into monochrome. TeshFxMonoColorFilter is a good way to learn how to use filters.
Prerequisites
It is assumed that the following environment is already in place, so if you do not have it yet, please follow the links to prepare it.
- Processing must be installed.
–>If not yet: Install Processing (Windows version, Mac version, Linux version) - The latest version of TeshFx must be installed.
→If you have not yet done so, install TeshFx.
Coding
The code below is a modified version of the DisplayTesh01 code. You may type from scratch to practice typing, or if you are lazy, you may copy DisplayTesh01 and add some lines to it.Save this as “Filter001”.
PImage image; // declare a variable "image"
import teshfx.filter.*; // declare to use TeshFxFilter
// Create a TeshFxMonoColorFilter and assign it to the variable "monoColorFilter"
TeshFxFilter monoColorFilter = new TeshFxMonoColorFilter();
void setup() {
size(480, 640); // Specify window size as 480x640
// Download the image specified by URL from the Internet and assign it to the variable "image".
image = loadImage("https://coding4.art/wp-content/uploads/2022/11/teshnakamura.jpg");
// Apply the filter to the image and reassign it to image.
image = monoColorFilter.filter(image);
}
void draw() {
image(image, 0, 0); // display "image"
}
void dispose() {
save("teshmono.png"); // save the image
}
When you execute this code, a handsome man converted to monochrome will be displayed.
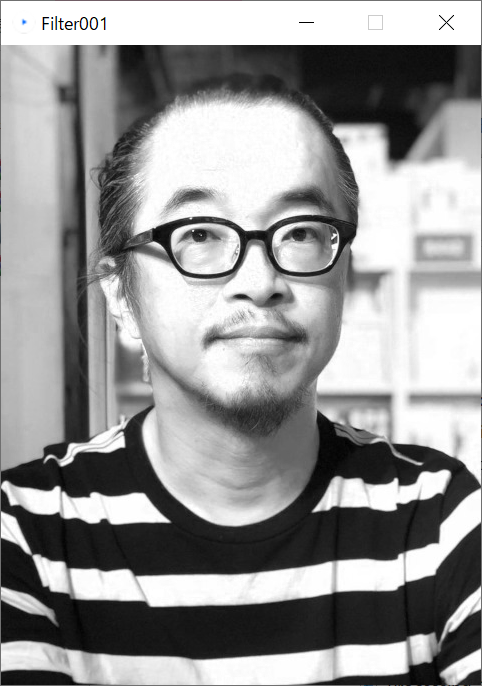
Explanation
The following is an explanation of what has been added since DisplayTesh01.
Line 3
This line declares the use of TeshFx filters. This is like a mantra. When you want to use TeshFx filters in the future, you need to write this.
Line 6
Create a TeshFxMonoColorFilter and assign it to the variable “monoColorFilter”.
Line 14
Applies the filter on the image, which was loaded at line 11.By typing “.” (period) in monoColorFilter followed by the function “filter”, the filter that monoColorFilter has is called.
The result of that filter function is again assigned to the image variable.
Remember that “=” (equal) meant “assign the value on the right side to the variable on the left side”. It does not mean “the left side equals the right side”.
Lines 21 – 23
The dispose function is called only once at the end of the program, so save the image here.
When you open the saved file after the program ends, you should see a monochrome image of a handsome man.
Summary
Here we learned how to use TeshFx filters by using TeshFxMonoColorFilter, which makes an image monochrome, as an example of TeshFx filters. The basics of how to use them are the same as in this article.
The procedure for using filters is as follows;
- Declares the use of a TeshFx filter. (import teshfx.filter.*;)
- Create a filter and assign it to a variable (TeshFxFilter xxx = new TeshFx—Filter();
- Call the filter function (xxx.filter())